Ipywidgets events
In [1]:
Copied!
import ipywidgets as widgets
import ipywidgets as widgets
In [2]:
Copied!
print(widgets.Button.on_click.__doc__)
print(widgets.Button.on_click.__doc__)
Register a callback to execute when the button is clicked. The callback will be called with one argument, the clicked button widget instance. Parameters ---------- remove: bool (optional) Set to true to remove the callback from the list of callbacks.
In [3]:
Copied!
button = widgets.Button(description="Click Me!", button_style="primary")
output = widgets.Output()
def on_button_clicked(b):
with output:
print("Button clicked.")
button.on_click(on_button_clicked)
widgets.VBox([button, output])
button = widgets.Button(description="Click Me!", button_style="primary")
output = widgets.Output()
def on_button_clicked(b):
with output:
print("Button clicked.")
button.on_click(on_button_clicked)
widgets.VBox([button, output])
Out[3]:
In [4]:
Copied!
print(widgets.Widget.observe.__doc__)
print(widgets.Widget.observe.__doc__)
Setup a handler to be called when a trait changes. This is used to setup dynamic notifications of trait changes. Parameters ---------- handler : callable A callable that is called when a trait changes. Its signature should be ``handler(change)``, where ``change`` is a dictionary. The change dictionary at least holds a 'type' key. * ``type``: the type of notification. Other keys may be passed depending on the value of 'type'. In the case where type is 'change', we also have the following keys: * ``owner`` : the HasTraits instance * ``old`` : the old value of the modified trait attribute * ``new`` : the new value of the modified trait attribute * ``name`` : the name of the modified trait attribute. names : list, str, All If names is All, the handler will apply to all traits. If a list of str, handler will apply to all names in the list. If a str, the handler will apply just to that name. type : str, All (default: 'change') The type of notification to filter by. If equal to All, then all notifications are passed to the observe handler.
First example¶
In [5]:
Copied!
buttons = widgets.ToggleButtons(
value=None,
options=["Show", "Hide", "Close"],
button_style="primary",
)
buttons.style.button_width = "80px"
html = widgets.HTML(
value='<img src="https://earthengine.google.com/static/images/earth-engine-logo.png" width="100" height="100">'
)
vbox = widgets.VBox([buttons, html])
vbox
buttons = widgets.ToggleButtons(
value=None,
options=["Show", "Hide", "Close"],
button_style="primary",
)
buttons.style.button_width = "80px"
html = widgets.HTML(
value='
'
)
vbox = widgets.VBox([buttons, html])
vbox
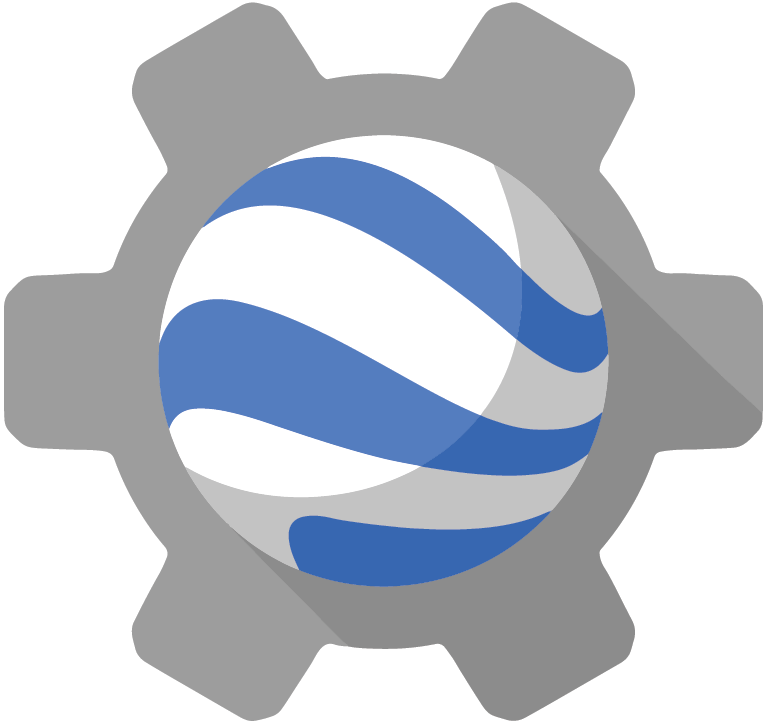
Out[5]:
In [6]:
Copied!
def handle_btn_click(change):
if change['new'] == 'Show':
vbox.children = [buttons, html]
elif change['new'] == 'Hide':
vbox.children = [buttons]
elif change['new'] == 'Close':
buttons.close()
html.close()
vbox.close()
buttons.observe(handle_btn_click, "value")
def handle_btn_click(change):
if change['new'] == 'Show':
vbox.children = [buttons, html]
elif change['new'] == 'Hide':
vbox.children = [buttons]
elif change['new'] == 'Close':
buttons.close()
html.close()
vbox.close()
buttons.observe(handle_btn_click, "value")
Second example¶
In [7]:
Copied!
dropdown = widgets.Dropdown(
options=["Landsat", "Sentinel", "MODIS"],
value=None,
description="Satellite:",
style={"description_width": "initial"},
layout=widgets.Layout(width="250px")
)
btns = widgets.ToggleButtons(
value=None,
options=["Apply", "Reset", "Close"],
button_style="primary",
)
btns.style.button_width = "80px"
output = widgets.Output()
box = widgets.VBox([dropdown, btns, output])
box
dropdown = widgets.Dropdown(
options=["Landsat", "Sentinel", "MODIS"],
value=None,
description="Satellite:",
style={"description_width": "initial"},
layout=widgets.Layout(width="250px")
)
btns = widgets.ToggleButtons(
value=None,
options=["Apply", "Reset", "Close"],
button_style="primary",
)
btns.style.button_width = "80px"
output = widgets.Output()
box = widgets.VBox([dropdown, btns, output])
box
Out[7]:
In [8]:
Copied!
def dropdown_change(change):
if change['new']:
with output:
output.clear_output()
print(change['new'])
dropdown.observe(dropdown_change, "value")
def dropdown_change(change):
if change['new']:
with output:
output.clear_output()
print(change['new'])
dropdown.observe(dropdown_change, "value")
In [9]:
Copied!
def button_click(change):
with output:
output.clear_output()
if change['new'] == "Apply":
if dropdown.value is None:
print("Please select a satellite from the dropdown list.")
else:
print(f"You selected {dropdown.value}")
elif change['new'] == 'Reset':
dropdown.value = None
else:
box.close()
btns.observe(button_click, "value")
def button_click(change):
with output:
output.clear_output()
if change['new'] == "Apply":
if dropdown.value is None:
print("Please select a satellite from the dropdown list.")
else:
print(f"You selected {dropdown.value}")
elif change['new'] == 'Reset':
dropdown.value = None
else:
box.close()
btns.observe(button_click, "value")
Last update:
2023-05-10